手写数字识别的神经网络实践
本示例代码为基于mnist数据集的手写数字识别的神经网络实践。示例用使用的手写数字板的识别图片可以参考https://code.5288z.com/zhangyuqing/AIPractice fc2目录下的pic文件夹下的相关图片。 整个内容包括4个文件,fc2_mnist_forward.py fc2_mnist_backward.py fc2_minist_test.py fc2_minist_app.py 其中前三个分别为该神经网络的执行和测试文件,在进行训练完毕后可以看到输出准确率可以达到98%以上,在重新运行fc2_mnist_app.py即可对响应的文件进行识别。下面分别是这四个文件的代码。
#coding=utf-8
#fc2_mnist_forward.py
#本文件是前向传播过程的代码
import tensorflow as tf
INPUT_NODE= 784
OUTPUT_NODE = 10
LAYER1_NODE = 500
def get_weight(shape,regularizer):
w = tf.Variable(tf.truncated_normal(shape,stddev=0.1))
if regularizer != None:
tf.add_to_collection('losses',tf.contrib.layers.l2_regularizer(regularizer)(w))
return w
def get_bias(shape):
b = tf.Variable(tf.zeros(shape))
return b
def forward(x,regularizer):
print regularizer
w1 = get_weight([INPUT_NODE,LAYER1_NODE],regularizer)
b1 = get_bias([LAYER1_NODE])
y1 = tf.nn.relu(tf.matmul(x,w1) + b1)
w2 = get_weight([LAYER1_NODE,OUTPUT_NODE],regularizer)
b2 = get_bias([OUTPUT_NODE])
y = tf.matmul(y1,w2) + b2
return y
#coding=utf-8
#fc2_mnist_backward.py
#本文件是反向传播过程的代码
import tensorflow as tf
from tensorflow.examples.tutorials.mnist import input_data
import fc2_mnist_forward
import os
BATCH_SIZE = 200
LEANRING_RATE_BASE = 0.1
LEANRING_RATE_DECAY = 0.99
REGULAERIZER = 0.0001
STEPS = 50000
MOVING_AVERAGE_DECAY = 0.99
MODEL_SAVE_PATH = "./model/"
MODEL_NAME = "mnist_model"
def backward(mnist):
x = tf.placeholder(tf.float32, [None, fc2_mnist_forward.INPUT_NODE])
y_ = tf.placeholder(tf.float32, [None, fc2_mnist_forward.OUTPUT_NODE])
y = fc2_mnist_forward.forward(x, REGULAERIZER)
global_step = tf.Variable(0,trainable=False)
ce = tf.nn.sparse_softmax_cross_entropy_with_logits(logits=y,labels=tf.argmax(y_,1))
cem = tf.reduce_mean(ce)
loss = cem + tf.add_n(tf.get_collection('losses'))
learning_rate = tf.train.exponential_decay(
LEANRING_RATE_BASE,
global_step,
mnist.train.num_examples / BATCH_SIZE,
LEANRING_RATE_DECAY,
staircase=True
)
train_step = tf.train.GradientDescentOptimizer(learning_rate).minimize(loss,global_step=global_step)
ema = tf.train.ExponentialMovingAverage(MOVING_AVERAGE_DECAY,global_step)
ema_op = ema.apply(tf.trainable_variables())
with tf.control_dependencies([train_step,ema_op]):
train_op = tf.no_op(name = 'train')
saver = tf.train.Saver()
with tf.Session() as sess:
init_op = tf.global_variables_initializer()
sess.run(init_op)
ckpt = tf.train.get_checkpoint_state(MODEL_SAVE_PATH)
if ckpt and ckpt.model_checkpoint_path:
saver.restore(sess,ckpt.model_checkpoint_path)
for i in range(STEPS):
xs,ys = mnist.train.next_batch(BATCH_SIZE)
_,loss_value,step = sess.run([train_op,loss,global_step],feed_dict={x:xs,y_:ys})
if i % 1000 == 0:
print "After %d training steps, loss on training batch is %g." % (step,loss_value)
saver.save(sess,os.path.join(MODEL_SAVE_PATH,MODEL_NAME),global_step=global_step)
def main():
mnist = input_data.read_data_sets("../data/",one_hot=True)
backward(mnist)
if __name__ == "__main__":
main()
#coding=utf-8
#fc2_mnist_test.py
#本文件为训练模型的数据集训练文件
import time
import tensorflow as tf
from tensorflow.examples.tutorials.mnist import input_data
import fc2_mnist_forward
import fc2_mnist_backward
TEST_INTERVAL_SECS = 5
def test(mnist):
with tf.Graph().as_default() as g:
x = tf.placeholder(tf.float32,[None,fc2_mnist_forward.INPUT_NODE])
y_ = tf.placeholder(tf.float32,[None,fc2_mnist_forward.OUTPUT_NODE])
y = fc2_mnist_forward.forward(x,None)
ema = tf.train.ExponentialMovingAverage(fc2_mnist_backward.MOVING_AVERAGE_DECAY)
ema_restore = ema.variables_to_restore()
saver = tf.train.Saver(ema_restore)
correct_prediction = tf.equal(tf.argmax(y,1),tf.argmax(y_,1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction,tf.float32))
while True:
with tf.Session() as sess:
ckpt = tf.train.get_checkpoint_state(fc2_mnist_backward.MODEL_SAVE_PATH)
if ckpt and ckpt.model_checkpoint_path:
saver.restore(sess,ckpt.model_checkpoint_path)
global_step = ckpt.model_checkpoint_path.split("/")[-1].split("-")[-1]
accuracy_score = sess.run(accuracy,feed_dict={x:mnist.test.images,y_:mnist.test.labels})
print "after %s training steps ,test accuracy = %g"%(global_step,accuracy_score)
else:
print "NO checkpoint file found"
time.sleep(TEST_INTERVAL_SECS)
def main():
mnist = input_data.read_data_sets("../data/",one_hot=True)
test(mnist)
if __name__ == "__main__":
main()
#coding=utf8
#fc2_minist_app.py
#本文件为最终生成的数据应用程序模型,下图中所运行的示例演示结果文件。
import tensorflow as tf
import numpy as np
from PIL import Image
import fc2_mnist_backward
import fc2_mnist_forward
def resotre_model(testPicArray):
with tf.Graph().as_default() as tg:
x = tf.placeholder(tf.float32,[None,fc2_mnist_forward.INPUT_NODE])
y = fc2_mnist_forward.forward(x,None)
preValue = tf.argmax(y,1)
variable_averages = tf.train.ExponentialMovingAverage(fc2_mnist_backward.MOVING_AVERAGE_DECAY)
variables_to_resotore = variable_averages.variables_to_restore()
saver = tf.train.Saver(variables_to_resotore)
with tf.Session() as sess:
ckpt = tf.train.get_checkpoint_state(fc2_mnist_backward.MODEL_SAVE_PATH)
if ckpt and ckpt.model_checkpoint_path:
saver.restore(sess,ckpt.model_checkpoint_path)
preValue = sess.run(preValue,feed_dict={x:testPicArray})
return preValue
else:
print "No checkpoint file found"
return -1
def pre_pic(picName):
img = Image.open(picName)
reIm = img.resize((28,28),Image.ANTIALIAS)
im_arr = np.array(reIm.convert('L'))
thredshold = 50
for i in range(28):
for j in range(28):
im_arr[i][j] = 255 - im_arr[i][j]
if im_arr[i][j] < thredshold:
im_arr[i][j] = 0
else:
im_arr[i][j] = 255
nm_arr = im_arr.reshape([1,784])
nm_arr = nm_arr.astype(np.float32)
img_ready = np.multiply(nm_arr,1.0/255.0)
return img_ready
def application():
testNum = input("input the number of test pictures:")
for i in range(testNum):
testPicPath = raw_input("the path of test picture:")
testPicArr = pre_pic(testPicPath)
preValue = resotre_model(testPicArr)
print "the prediction number is:",preValue
def main():
application()
if __name__ == '__main__':
main()
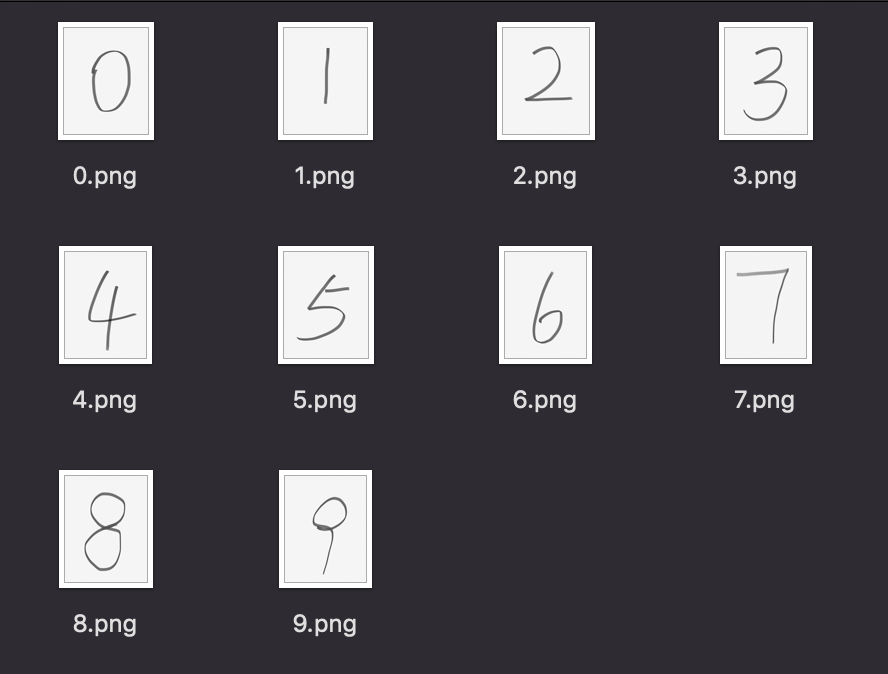
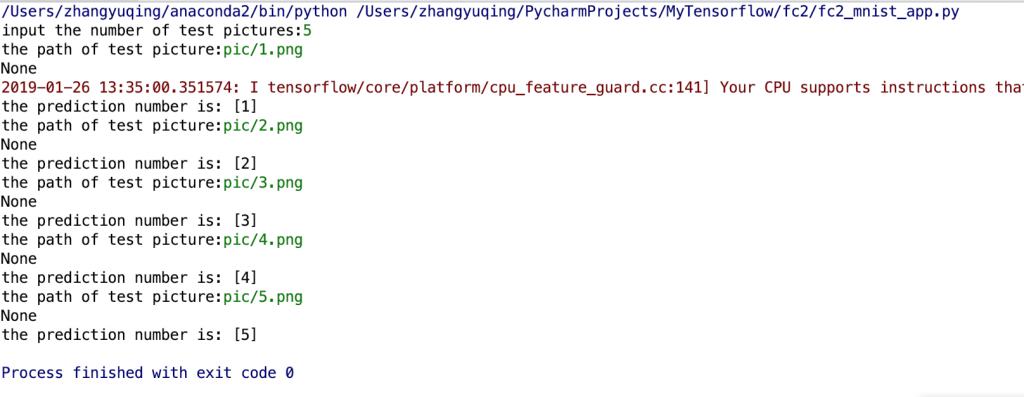